Angular has a powerful dependency injection system. Learn how to use it so that your code complies with the Dependency Inversion Principle.
Dependency Inversion in Angular
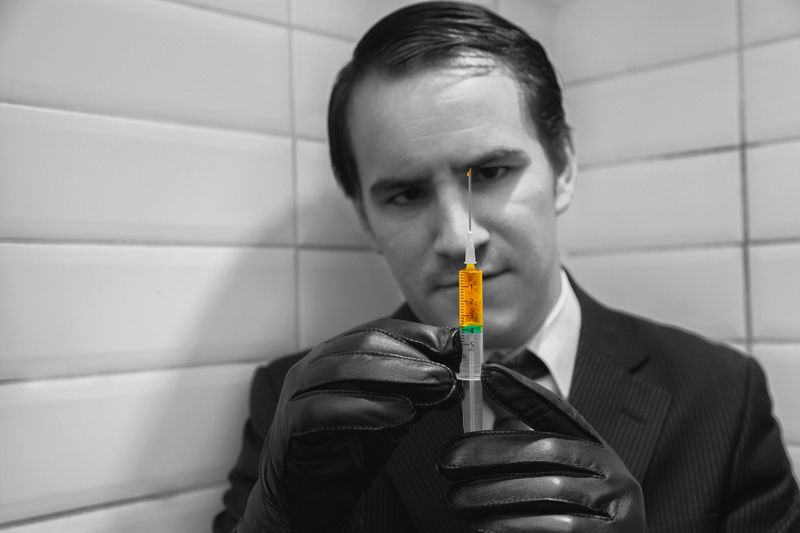
Angular has a powerful dependency injection system. Learn how to use it so that your code complies with the Dependency Inversion Principle.
If you're getting a little more advanced in your JavaScript, you may have started using the [Symbol.iterator] to make your objects iterable. Using a generator function allows for much more idiomatic looking code and saves you from having to return an object that conforms to the iterable protocol.
Reading a number like 1000000000000 on a line of code may be a breeze for your computer but is next to impossible for a human to do without getting a headache or nose bleed. What can we do to make large numbers more readable to the human eye?
BigInt is a relatively new addition to JavaScript and many aren't familiar with it. This was my case until recently. While working on the Closest Number puzzle on condingame.com, I found myself having to deal with numbers of sizes as big as 10^1000.
Accessing items in a 2D array can sometimes be a pain. I have created an Array2D class that extends Array and eases some of that pain.